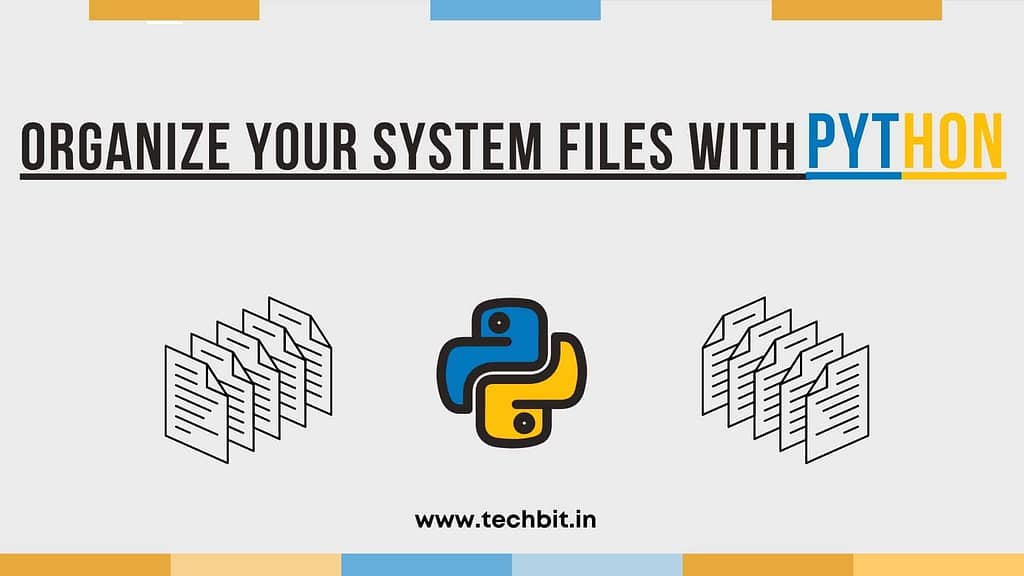
Hello, So this one is a real quick python script that is surely gonna save a lot of time. We will be able to declutter any folder and move particular files (based on the file extension) to any destination folder that you want. This is just a basic script, and a lot of features can be added to it.
Okay Let’s start this tutorial:
- So first of all, we need to import some modules to go further.
import os
import shutil
from pathlib import Path
OS module is a very useful module that provides Operating System-related functions to either creating or removing a directory/file, checking the current directory, and changing it, etc. Shutil is also a powerful module that lets you perform high-level functions. It can automate functions like copying, creating, and removal of files and folders. The pathlib module lets you work with filesystem paths. It works on classes which is an OOPS concept(Object Oriented Programming).
- In this tutorial, I have used my “Downloads” Folder to declutter, you can use any folder and give the path to it.
downloads_path = str(Path.home() / "Downloads")
# print(os.path.isdir(downloads_path))
# You can print the path, just to make sure that the path you have given is working and you are good to go ahead.
Note: You can even get to the home directory with the ‘os module’ itself. For that, the code will look like this:
from os.path import expanduser
home = expanduser("~")
- Just give the sourcepath and sourcefiles parameters. This will fix your source folder and will further list all the files present there.
sourcepath = downloads_path
sourcefiles = os.listdir(sourcepath)
- Now we have to make a destination folder to which our files from the source folder will get moved.
# give the path where you want your destination folder to be
path = "F:\\TECHBIT\\songs"
# mkdir for making a diirectory
os.mkdir(path)
# setting the destination path as the path, where we just made a new folder
destinationpath= path
- Lastly, we have to loop it up for all the files in the source folder. You can pass multiple arguments here to customize the code according to your need. I need to move my “.mp3” files from the “Downloads” folder to a folder named “songs” in my F drive. So accordingly, I chose the file type as “.mp3”.
- The shutil module first works with joining the source path and the file and then moving the file with the “.mp3” file type to the destination path.
# give the path where you want your destination folder to be
for file in sourcefiles:
if file.endswith('.mp3'):
shutil.move(os.path.join(sourcepath, file),
os.path.join(destinationpath, file))
**You can run the same code for any file extension, just replace the “.mp3” with your desired file extension.
Here’s the complete code with the pathlib module:
import os
import shutil
from pathlib import Path
downloads_path = str(Path.home() / "Downloads")
sourcepath = downloads_path
sourcefiles = os.listdir(sourcepath)
path = "F:\\TECHBIT\\songs"
os.mkdir(path)
destinationpath= path
for file in sourcefiles:
if file.endswith('.mp3'):
shutil.move(os.path.join(sourcepath, file),
os.path.join(destinationpath, file))
Alternatively, you can just use the OS module and Shutil. It will work just the same. Here’s the complete code for that:
import os
import shutil
from os.path import expanduser
home = expanduser("~")
sourcepath = home
sourcefiles = os.listdir(sourcepath)
path = "F:\\TECHBIT\\songs2"
os.mkdir(path)
destinationpath= path
for file in sourcefiles:
if file.endswith('.mp3'):
shutil.move(os.path.join(sourcepath, file),
os.path.join(destinationpath, file))
That’s all for now. I hope it will help you somewhere and it was worth your time. Do share your feedback and also your approach to the same problem.
Check out my latest articles here:
2 Comments
Aathira · July 9, 2021 at 8:06 am
Nice explanation
Vaishali_Rastogi · July 9, 2021 at 8:30 am
Thanks, Aathira.