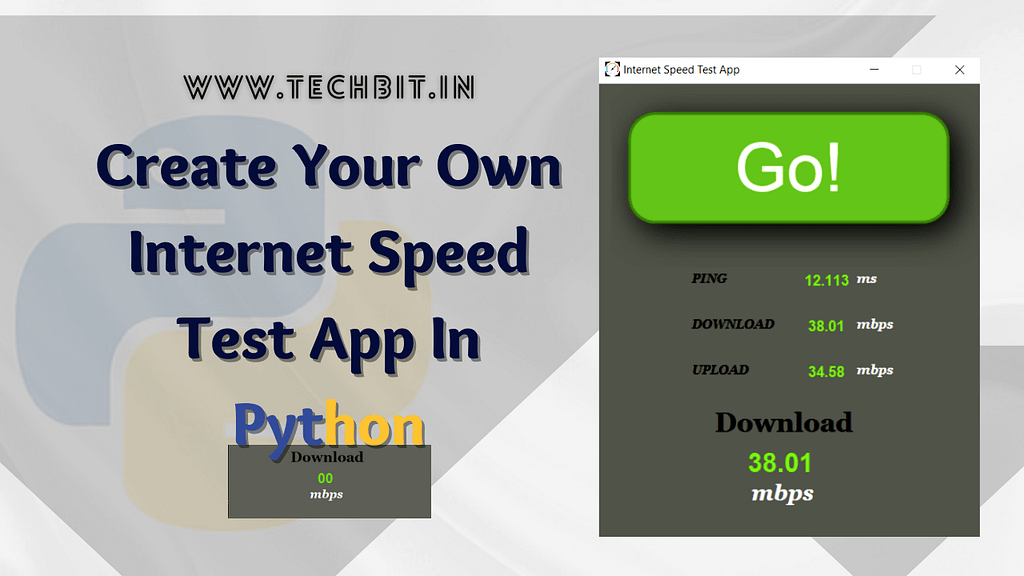
Our Objective: To create our own Internet Speedtest App using Speedtest Python
Python is the top-most programming language is a very powerful and extensively used language. Because it is a general-purpose language, it has applications in various domains. Python has not left any domain untouched, be it Game Development, Web Development, Machine Learning, Data Science, GUI Applications, and many more.
According to the TIOBE Programming Community index, Python not only retained the top spot of the most popular programming languages but also saw a 3.95% increase in usage from March 2021 to 2022.
Coming straight to the topic, sometimes running the internet speed test becomes so crucial to know the current state of our internet. So we are just going to build the same in this tutorial. We are focusing to create a GUI-based Internet Speedtest Application. The language is of course Python and the libraries we are going to use are Speedtest and Tkinter.
Table of Contents
Speedtest Python Introduction
Speedtest is a Python library that is used to check the internet bandwidth using speedtest.net. It is built to run on a command-line interface. It was first released in 2013, and now version 2.1.3 is running.
Installing Speedtest
Since this library is not a built-in library, we have to install it using PIP. To install speedtest, open your command prompt and type in the following command:
pip install speedtest-cli
Python Tkinter Introduction
Tkinter is the other library that we need for this project. There are many GUI-based frameworks available for python, but Tkinter is the only framework that’s built into the Python standard library. Tkinter helps in creating the graphical user interface for desktop-based applications.
Installing Tkinter
It is a built-in library as I already mentioned. However, if by any chance we are not able to find it in our pre-installed python using pip, we can install it using the following command:
pip install tk
Our Approach To Code This Python Project
As we have already discussed that we’ll be needing these two libraries pre-installed to get started:
- speedtest-cli
- tkinter
Speedtest will help us in achieving our objective by telling us the ping, upload, and also download speed of our Internet connection. Whereas Tkinter will help us in building a GUI(Graphical-User-Interface) platform through which we can run this internet speed test provided by Speedtest-CLI Library.
The work is very simple and easily achievable by only using the speedtest library. But it won’t look as easy and attractive as to what using Tkinter will make it look like. The motto of giving a Graphical outlook to the otherwise basic program is what the fun part is all about. So let’s quickly dive into that.
Folder Structure
To get started we first need to understand the folder structure real quick. So in my working directory, I have made one folder named “Internet Speedtest App”. Following are the things that I have in that folder:
- a file with the name and the extension as “index.py“
- an icon image (to be used as the favicon in our app)
- and another image that says “Go”, which we will need when coding the GUI part of our app.
How To Use Speedtest Python Library?
I have provided the complete code below. The code on execution will open up an Internet Speed Check Application. But apart from Tkinter or giving it a GUI, let’s first understand how we can use it and what all we can do with Speedtest Library:
- We will first import Speedtest library. For that write the following command in your index.py file:
import speedtest
- Now we have speedtest library avaliable to us in the current project. Then we have created an instance of Speedtest and named it as “test”.
test = speedtest.Speedtest()
- Using speedtest, we can check the upload speed , the download speed and the ping. So we are just going to do that. The library has methods for getting the all said values. So we are going to make use of the methods and store the resulting speed in the corresponding variables.
- Check for Download Speed:
d_test = test.download()
- Check for Upload Speed:
u_test = test.upload()
- Check for Ping:
ping = test.get_servers([])
- As we have stored the value of download, upload speed and the ping in their respective variables. We are now gonna be printing them to see the result:
print("Your Download speed is:", d_test)
print("Your Upload speed is:", u_test)
print("Your Ping is:", ping)
The outcome when we run our code:
Your Download speed is: 41287200.16963193
Your Upload speed is: 11183324.329613607
Your Ping is: 16.45
Coding Our App using Speedtest Python and Tkinter Modules
Here is the complete code with the GUI interface which integrates Speedtest methods and tkinter’s GUI methods. We have used the above code in a form of a function that gets triggered once the user clicks on the “Go” Button. The rest of the application is static and is built solely using Tkinter.
from tkinter import * import speedtest root = Tk() root.title("Internet Speedtest App") root.geometry("420x500") root.resizable(False, False) root.configure(bg="#5D5F56") def Check(): test = speedtest.Speedtest() Uploading = round(test.upload() / (1024 * 1024), 2) upload.config(text=Uploading) downloading = round(test.download() / (1024 * 1024), 2) download.config(text=downloading) Download.config(text=downloading) servernames = [] test.get_servers(servernames) ping.config(text=test.results.ping) #icon img_icon = PhotoImage(file="Logo.png") root.iconphoto(False, img_icon) #images button = PhotoImage(file="start.png") Button = Button(root, image=button, activebackground="#5D5F56", bd=0, bg="#5D5F56", cursor="hand2", command=Check) Button.pack(padx=(15, 0)) #Labels Label(root, text="PING", font="georgia 10 bold italic", bg="#5D5F56").place(x=100, y=202) Label(root, text="DOWNLOAD", font="georgia 10 bold italic", bg="#5D5F56").place(x=100, y=252) Label(root, text="UPLOAD", font="georgia 10 bold italic", bg="#5D5F56").place(x=100, y=302) Label(root, text="ms", font="georgia 10 bold italic", bg="#5D5F56", fg="white").place(x=280, y=202) Label(root, text="mbps", font="georgia 10 bold italic", bg="#5D5F56", fg="white").place(x=280, y=252) Label(root, text="mbps", font="georgia 10 bold italic", bg="#5D5F56", fg="white").place(x=280, y=302) Label(root, text="Download", font="georgia 22 bold", bg="#5D5F56").place(x=125, y=350) Label(root, text="mbps", font="georgia 18 bold italic", bg="#5D5F56", fg="white").place(x=165, y=430) ping = Label(root, text="00", font="arial 12 bold", bg="#5D5F56", fg="#76DC0F") ping.place(x=250, y=215, anchor="center") download = Label(root, text="00", font="arial 12 bold", bg="#5D5F56", fg="#76DC0F") download.place(x=250, y=265, anchor="center") upload = Label(root, text="00", font="arial 12 bold", bg="#5D5F56", fg="#76DC0F") upload.place(x=250, y=315, anchor="center") Download = Label(root, text="00", font="arial 22 bold", bg="#5D5F56", fg="#76DC0F") Download.place(x=200, y=415, anchor="center") root.mainloop()
Here is the outcome of this code:
So that’s it with the code and the article. It was this easy-to-use Speedtest library and Tkinter made it this easy to make an app out of it. You are free to design the app according to your liking. Make sure to give it a try.
I hope this tutorial has helped. One can always play around with both the libraries and explore more features yourself. Any feedback or suggestion/doubt is always welcome. Comment down below if you have any. Thank you for reading the article 🙂
Let’s get connected at our Social Media Handles:
Make sure to look at our other python projects too:
- CSS 01: How To Use Bulma CSS To Style Your Next Login Form?
- How To Easily Find Image Size Using Python? (3 Methods)
- This is the Ultimate Python Code to Turn Any Image To ASCII Art
- Easily Code A Weather App In Python Using Tkinter
- 8 Best Python Books for Beginners and Advanced Programmers
- How to Code a Simple GUI Calculator in Python using Tkinter
- Easy Script to Make Your Own Youtube Video Downloader with Python
2 Comments
kaca tempered · March 23, 2022 at 9:37 am
I every time emailed this website post page to all my contacts, for the
reason that if like to read it after that my contacts will too.
Vaishali Rastogi · March 23, 2022 at 6:38 pm
I highly appreciate it, thank you!